FLASH Programming in STM32
Master internal Flash programming on STM32: page-based (F1/M0) & sector-based (F4/H7), with erase‑write‑read HAL functions and code examples. The project is available for download at the end of the post.
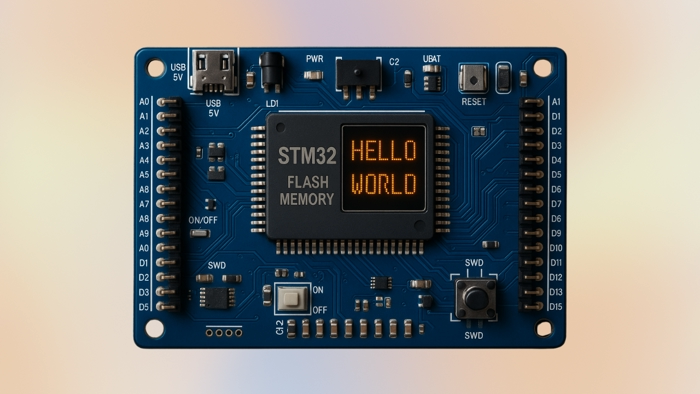
This tutorial is divided into two sections for clarity and relevance.
The first section focuses on STM32 microcontrollers with page-based flash memory, such as those from the Cortex-M3 and Cortex-M0 series.
The second section covers microcontrollers with sector-based flash memory, commonly found in higher-end devices like the STM32F4 and H7 series.
H7 Series users can get the files from https://github.com/controllerstech/STM32/tree/master/FLASH_PROGRAM/H7%20SERIES
Introducing STM32 Flash Memory
The Flash memory in STM32 microcontrollers is a type of non-volatile memory used to store the program code, configuration data, and user-defined variables that must persist across power cycles. Unlike SRAM, Flash retains data even when the device is turned off, making it essential for embedded applications.
STM32 Flash memory is organized in different ways depending on the microcontroller series:
- Page-based memory (e.g., STM32F1, STM32L0, Cortex-M0/M3 series)
- Sector-based memory (e.g., STM32F4, STM32H7 series)
Flash memory can be accessed in the following ways:
- Read access – Directly read like normal memory using pointers.
- Write access – Requires unlocking the Flash control register, erasing a page or sector, and then writing data.
- Erase operations – Can be page-wise or sector-wise depending on the device.
- Bootloader access – STM32 also supports writing to Flash via built-in bootloaders (UART, USB, etc.).
The STM32 HAL library provides functions like HAL_FLASH_Program()
and HAL_FLASHEx_Erase()
to simplify flash operations safely and reliably.
Features of STM32 Flash Memory:
- Non-Volatile Storage
Retains data even after power-off, ideal for storing firmware, calibration data, and settings. - Page or Sector Erase Support
Enables partial memory erasure, making it flexible for dynamic data updates and firmware over-the-air (OTA) applications. - Fast Read Access
Flash is mapped to the instruction bus, allowing fast code execution directly from memory (also supports prefetch and cache in higher series). - Protection and Security Options
Includes read/write protection, PCROP (Proprietary Code Readout Protection), and option bytes to safeguard critical data and firmware.
PAGE-BASED FLASH MEMORY
For the first half, I am using STM32F103 microcontroller, and you can see the memory distribution in the picture below.
As you can see above that the main memory (Flash memory) is distributed in 128 pages. Each page is of 1 KB, thus making the total memory of 128 KB
Now always remember that we should start programming as lower as possible in the flash memory. This is because the start of the flash is already allocated to the program, that is being executed on your controller right now. I have explained it properly in the video, you can check that out in the end.
Each page can hold 1024 Bytes of data, and i don’t have much data to write, so I will choose the last page of the flash memory, i.e. 0x0801FC00 – 0x0801FFFF
The Code
Write Data to FLASH
Below is the function to write the data into the Flash.
uint32_t Flash_Write_Data (uint32_t StartPageAddress, uint32_t *Data, uint16_t numberofwords);
The function Flash_Write_Data
takes the following parameters
- @ StartPageAddress is the address of the Start page, or memory in the page, from where you want to start writing the data.
- @ Data is the pointer to the 32 bit data array, that you want to write into the flash.
- @ numberofwords is the number of words, that you want to write in the memory.
The function is very big to be able to explain here. But here are few important hings that it does in order to write the data into the given memory location.
- Flash will be Unlocked to make modifications.
- Based on the number of words and start page address, it will calculate the number of pages required to store that data into.
- Next, the required number of pages will be erased, and the new data will be written into them.
- Flash will be Locked again.
Read Data from FLASH
Below is the function to read the data from the Flash.
void Flash_Read_Data (uint32_t StartPageAddress, uint32_t *RxBuf, uint16_t numberofwords);
The function Flash_Read_Data
reads the data from the flash. It takes the following parameters
- StartPageAddress is the Start address of the page, from where you want to start reading the data.
- RxBuf is the address of the 32 bit array, where you want to store the data.
- numberofwords is the number of words that you want to read from the memory.
Main Function
char *data = "hello FLASH from ControllerTech\
This is a test to see how many words can we work with";
uint32_t data2[] = {0x1,0x2,0x3,0x4,0x5,0x6,0x7,0x8,0x9};
uint32_t Rx_Data[30];
char string[100];
int number = 123;
float val = 123.456;
float RxVal;
Flash_Write_Data(0x08004410 , (uint32_t *)data2, 9);
Flash_Read_Data(0x08004410 , Rx_Data, 10);
int numofwords = (strlen(data)/4)+((strlen(data)%4)!=0);
Flash_Write_Data(0x08004810 , (uint32_t *)data, numofwords);
Flash_Read_Data(0x08004810 , Rx_Data, numofwords);
Convert_To_Str(Rx_Data, string);
Flash_Write_NUM(0x08005C10, number);
RxVal = Flash_Read_NUM(0x08005C10);
Flash_Write_NUM(0x08012000, val);
RxVal = Flash_Read_NUM(0x08012000);
Here I am writing 4 different data types to the different locations in the memory.
After we perform the read, the same data will be available, and this indicates that the write and read was successful.
Result
Check out the Video Below
SECTOR-BASED FLASH MEMORY
Some microcontrollers have memory distributed in Sectors.
In my STM32F446RE, there are 7 sectors and the size is varying between them. Similarly, some microcontrollers can have 11, 15 or 23 sectors. This code will cover all these types, irrespective of the number of sectors the controller have.
IMPORTANT
If the memory for the controller is arranged in dual banks, like Bank1 or Bank2, by defaults these Banks are disabled and the memory uses only single Bank addressing. Check the sector addresses in the single Bank distribution.
Also, if the sector sizes are different than as shown in the picture above, All you need to do is, define new sectors as according to your device datasheet in the function static uint32_t GetSector(uint32_t Address)
The Code
Write Data to FLASH
uint32_t Flash_Write_Data (uint32_t StartSectorAddress, uint32_t *Data, uint16_t numberofwords)
{
static FLASH_EraseInitTypeDef EraseInitStruct;
uint32_t SECTORError;
int sofar=0;
/* Unlock the Flash to enable the flash control register access *************/
HAL_FLASH_Unlock();
/* Erase the user Flash area */
/* Get the number of sector to erase from 1st sector */
uint32_t StartSector = GetSector(StartSectorAddress);
uint32_t EndSectorAddress = StartSectorAddress + numberofwords*4;
uint32_t EndSector = GetSector(EndSectorAddress);
/* Fill EraseInit structure*/
EraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS;
EraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3;
EraseInitStruct.Sector = StartSector;
EraseInitStruct.NbSectors = (EndSector - StartSector) + 1;
/* Note: If an erase operation in Flash memory also concerns data in the data or instruction cache,
you have to make sure that these data are rewritten before they are accessed during code
execution. If this cannot be done safely, it is recommended to flush the caches by setting the
DCRST and ICRST bits in the FLASH_CR register. */
if (HAL_FLASHEx_Erase(&EraseInitStruct, &SECTORError) != HAL_OK)
{
return HAL_FLASH_GetError ();
}
/* Program the user Flash area word by word
(area defined by FLASH_USER_START_ADDR and FLASH_USER_END_ADDR) ***********/
while (sofar<numberofwords)
{
if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, StartSectorAddress, Data[sofar]) == HAL_OK)
{
StartSectorAddress += 4; // use StartPageAddress += 2 for half word and 8 for double word
sofar++;
}
else
{
/* Error occurred while writing data in Flash memory*/
return HAL_FLASH_GetError ();
}
}
/* Lock the Flash to disable the flash control register access (recommended
to protect the FLASH memory against possible unwanted operation) *********/
HAL_FLASH_Lock();
return 0;
}
- Flash_Write_Data will write data to the given memory location.
- It will calculate the start sector number based on the address given in the parameter
- then, the sector address of the sector where the data is going to end
- and finally the sector number of the last sector
- It will than erase the required number of sectors and program it with the new data
Read Data from FLASH
void Flash_Read_Data (uint32_t StartSectorAddress, uint32_t *RxBuf, uint16_t numberofwords)
{
while (1)
{
*RxBuf = *(__IO uint32_t *)StartSectorAddress;
StartSectorAddress += 4;
RxBuf++;
if (!(numberofwords--)) break;
}
}
Flash_Read_Data will read the given amount of words from the memory location, and save it in the RxBuf
Main Function
char *data = "hello FLASH from ControllerTech\
This is a test to see how many words can we work with";
uint32_t data2[] = {0x1,0x2,0x3,0x4,0x5,0x6,0x7,0x8,0x9};
uint32_t Rx_Data[30];
char string[100];
int number = 123;
float val = 123.456;
float RxVal;
Flash_Write_Data(0x08004100 , (uint32_t *)data2, 9);
Flash_Read_Data(0x08004100 , Rx_Data, 10);
int numofwords = (strlen(data)/4)+((strlen(data)%4)!=0);
Flash_Write_Data(0x08008100 , (uint32_t *)data, numofwords);
Flash_Read_Data(0x08008100 , Rx_Data, numofwords);
Convert_To_Str(Rx_Data, string);
Flash_Write_NUM(0x0800C100, number);
RxVal = Flash_Read_NUM(0x0800C100);
Flash_Write_NUM(0x0800D100, val);
RxVal = Flash_Read_NUM(0x0800D100);
Here I am writing 4 different data types to the different locations in the memory.
After we perform the read, the same data will be available, and this indicates that the write and read was successful.
Result
Check out the Video Below
PROJECT DOWNLOAD
Info
You can help with the development by DONATING Below.
To download the project, click the DOWNLOAD button.
🙏 Support Us by Disabling Adblock
We rely on ad revenue to keep Controllerstech free and regularly updated. If you enjoy the content and find it helpful, please consider whitelisting our website in your ad blocker.
We promise to keep ads minimal and non-intrusive.
Thank you for your support! 💙
Hello Thanks for the tutorial.
Writing and reading is working for me, BUT when i then try to program again my MCU after a writing the prog/debugger can complete anymore the interaction.
I see error
Error message from debugger back end:
Error finishing flash operation
Error finishing flash operation
in order to be able to program/debug again i have to make a full erase and comment the writing function and reprogram.
I’m using a stm32F439 ZI.
Thanks again!
send email at chayaporngaraipoom@gmail.com
Hey i have similar issue and tried to email but the email does ot exist
Thanks for the great tutorial. Helped me a lot. I noticed one minor bug in Flash_Read_Data. It should be
if (!(–numberofwords)) break;
instead of
if (!(numberofwords–)) break;
Otherwise there will be one additional loop run that might cause problems.
Thanks for this great tutorial. Helped me a lot. I noticed one minor bug in Flash_Read_Data. The line
if (!(numberofwords–)) break;
should be prefix decrement. So it should be changed to
if (!(–numberofwords)) break;
Otherwise there is one extra loop run.
I’m using your code from Github and I’m using STM32F103RC as well but the code didn’t work. I’m using the address 0x08005C10 and 0x08005D10. Write NUM function did not actually wrote anything into the flash (as I checked with the monitor it didn’t) and read NUM function only returns 0.
Hello Sir,
We are using STM32L432 and we are always getting error code as 128 that is FLASH_FLAG_MISERR. There are 2 changes done in our software.
EraseInitStruct does not have PageAddress element
EraseInitStruct.PageAddress = StartPage;
This was updated as
EraseInitStruct.Page = 127;
This error FLASH_FLAG_MISERR is appeared as part of the Page erase process. the following function output was 1.
status = FLASH_WaitForLastOperation((uint32_t)FLASH_TIMEOUT_VALUE);
The following error output was 128
error = (FLASH->SR & FLASH_FLAG_SR_ERRORS);
Do we have the example software for page erase for STM32L432 please?
Show less
Hello Sir,
We could write once and we are anot able to erase and update the last page. It looks like the flash is write protected. I meant we can compile and flash the chip. but we are not able erase the last page in our application software. How do we know the flash is write protected and How to clear the write protect before page erase? Could you please help us out?
I’m working on STM32H750 with FreeRTOS and code not work, the function HAL_FLASHEx_Erase error, the function run to FLASH_WaitForLastOperation and return HAL_TIMEOUT.
When i comment:
if (HAL_FLASHEx_Erase(&EraseInitStruct, &SECTORError) != HAL_OK)
{
return HAL_FLASH_GetError();
}
program work fine.
What the happen, I’m a little confused here. Do you have any suggest for me.
Hello,
I would like to know, Why a STM32 board binary code doesn’t run after I just flashed back to it of what I just red from the same board by using STM32 Cube Programmer?
Hi. It’s awesome tutorial. I have one question here.) What will happen to data in flash if power off and on
2) what will happen, if we flash again with read only function in main loop
Hi! Awesome tutorial, thanks for proper insight 🙂
Short question, why do you need to typecast again the pointer to array (uint32_t *) in the function Flash_Write_Data?
I am a little confused here 😀
Thanks!!
There is no need for that. I was testing with some strings (chars), so I might have done it because of that.
Hi,
I’m using STM32F429. I found your flash_sector_f4.c perfectly suited to my initial needs of storing binary data in a sector of flash. Thank you!
Now I’d like to be able to overwrite my running firmware with a new application after I’ve gotten it into RAM. I can’t use the built in bootloader. Is there an example of that anywhere? Thanks, Mike
You should check out this video series https://youtu.be/OkUQ3iMmiYQ
Hello,
I use STM32H725.
I need to implement Flash_Write_Data() at Low Level , without HAL .
Does anyone have low-level realization experience Write flash?
hello I have a problem
I can’t find the Flash_Write_NUM and Flash_Read_NUM functions
Para stm32F030C8, sua memória flash é páginas, mas quantas?
gracias
Hello
I have a question, and how can I get the free memory space I have left?
is there a HAL command?
Greetings from Colombia, your projects have helped me a lot
I’m work with the STM32F429 and stmcubeide. I’ve a error – conflicting types for ‘Flash_Write_Data’- when call a function uint32_t Flash_Write_Data (uint32_t StartSectorAddress, uint32_t *Data, uint16_t numberofwords)
{
int numofwords = (strlen(data)/4)+((strlen(data)%4)!=0);
Flash_Write_Data(0x08008100 , (uint32_t *)data, numofwords);
Flash_Read_Data(0x08008100 , Rx_Data, numofwords);
sprintf(teste_data2_char, “%d”, Rx_Data);
HAL_UART_Transmit(&huart1, (uint8_t *) teste_data2_char, strlen(teste_data2_char),500);
You are using older one.
Click the download button, it will take you to the github.
Download according to your controller
.
Hello sir Thank you very much for this code. I am working with the STM32G474RE. With your code I manage to write it but the delete is still not possible, what should I change or add? Thanks
which one did you used ?
hello dear
I used the “Flash Page Type”.
I do not know why I manage to write, but Erasing does not work, I always have to go through STM32 ST-LINK utility to be able to delete. could you please help me ?
The memory division in G4 is different, so you can’t use it directly.
It’s further divided into BANKS.
Maybe you can disable the banks and force the controller to use single BANK.
Also check the page size during debug.
unfortunately I’m only a beginner in this field, I don’t know how to force my controller to use the single Bank. Thank you once again for your time and your answers
Check your reference manual to know how to do that.
I am also working with STM32G474RE. Could you please share your insights or maybe even your code how you managed to write to the flash? Thank you so much!
hello sir .. i am new to this 32 bit this controller. i am writing code for stm32f207. while writing the flash ,controller gets stops in erase function, what is the problem , i am using base address is sector a10 and end address is sector11 .actually my flash data is 150 bytes only
If this FLASH_PAGE would be used with STM32L4 series where page size is 64bits, how big of a modification would this need? Thank you.
I also want to use the program for stm32L4 series please help as i got error while writing in similar fashion
Thanks to the Admin for sharing the Knowledge! Did someone manage to change the code for a STM32L4xx, in my case L452 (512x1Kb Pages)?
i write this code in keil MDK but has error for “(strlen(DATA_32)”
ERROR text:
(..\Src\FlASH_PAGE.c(109): error: #167: argument of type “uint32_t *” is incompatible with parameter of type “const char *”)
change the type inside strlen function
How can I use this code if my data is uint8_t?
I cant use the read function for ota purposes because in your function it only breaks when there is an empty cell. otherwise it runs until the end!!!!!!!!!!!!!!!!
therefore I added size parameter.
Hi!
I want to report some bug in FLASH_SECTOR. During saving I was getting a hardfault. The problem is that function HAL_FLASH_Program() expects the address of the variable to be saved. And you send a value DATA_32[sofar]. It should be the address: &DATA_32[sofar]. Great job again 🙂
Greetings!
F.Rak
In FLASH_SECTOR read function should be some other stop condition. Your stop condition is when you find 0xffffffff value in the array where you store read data. When this array is defined as global, the momory is alocated just for given array size. So when you want to read 2 bytes then you define array for 2 elements. In memory is alocated only 2 cells. After this 2 cells, other program data exist. So when you read in flash_sector_read more then 2 bytes you will plow the memory. This function should get one more parameter that is number of variables to read. Then one if statement it works much better.
Regards,
F.Rak
yeah ofcouse we can do that. The point in writing the 0xffffffff was that it will read the data of unknown length. But if you already know the length, then it should be easy just like you said.
When I write to the Flash I get memory protection and page alignment errors
memory protection might be turned on. Read your reference manual on how to remove it
Hi Sir.
How to find start page address ? In datasheet show page 127 address. I want to know another start page address.
They all are 1Kb in size. Just multiply 1024 to find the address.
For eg- 4*1024 = 4096 (0x1000) is the start address of page 4
127 *1024 = 130048 (0x1FC00) is the start address of page 127
Oh… Thanks. May I dump.
ex- 111*1024=113664(0x1bc00) right?
Thanks again.
yes. Make sure your flash size is 128 KB. Some bluepills have 64 KB
I copy your library and your code. Then string flash in 0x1FC00 that my bluepills have 128KB right. I plan to make my custom pcb with STM32F103C8T6 chip on it for prototype product. I think to be sure my code is write on it have 64KB for safe my product. Many THANKS.
I’m using Black Pill when i write to memory address it write and read quite well. But if i write in a loop for example i want to write to 0x08000400 and 0x08000400 the last written address is only stored in flash others are not stored why it’s happening? It’s urgent. Thank you
i didn’t understand the issue properly.. you have mentioned the same address twice in your comment
I’m sorry for my bad explanation here is the sample code..
After running this code data is stored only at this address 0x08006050 but during the iteration i can see that data is being stored by calling Read_Flash function and the output is this
14:10:19.482 -> Hello World
14:10:23.535 -> Hello World 1
14:10:25.564 -> Hello World 2
14:10:27.595 -> Hello World 3
But if i check the memory location using STM32CubeProgrammer i can only see last address data is stored in flash…
and the output is this
14:22:06.325 ->
14:22:08.329 ->
14:22:10.322 ->
14:22:12.354 -> Hello World 3
I don’t know what causing this..
you can not write data like that. You are trying to increase the address every few bytes. Check the page size of your controller in reference manual. Let’s assume it’s 1 KB. That means you can write once within that 1 KB size. If you try to write with an offset of 4 bytes, it will anyway erase the entire page and then write to that address.
I am using this concept to stm32f030f4 the value is uploaded to the flash memory address correctly and stored even though when the power is off but it does not reflect on the project kit when i reset the stm it works as per the coding logic it does not get the address value
thanks in advance
Thank you sir.
Hi,
i am using stm32H753 it has 2 memory banks BANK1 & BANK2 with 7 sectors each, and its a cortex-m7 controller,
i tried to use our program.it is not working
I checked the reference manual. Looks like there is a new memory system there. I need your project in order to modify it according to BANK type distribution. Contact me on that telegram group linked on the top right corner of website, or send a mail
Hi, I am using STM32H753 and i have same problem. How can i fix the problem? Can you help me please.
I will release an update for H7 series in a day or 2
Have you released the update for H7 series board? If yes, please share the link
Check the page again. It’s on the top
Thanks for the update. When i used the code for H7 series i got below error:
In function ‘Flash_Write_Data’:
../Drivers/STM32H7xx_HAL_Driver/Inc/Legacy/stm32_hal_legacy.h:425:39: error: ‘FLASH_VOLTAGE_RANGE_3’ undeclared (first use in this function);
I am using STM32H7A3 Nucleo board. I could not find out a definition for FLASH_VOLTAGE_RANGE_3 in my code. Please help me..
Try to comment out that line, and check.
Also make sure that the Sectors and Banks are defined properly in the flash_sector_h7.c file
Flash_Page.c file is written by you. Or It is in built HAL library file?
I wrote it, but took the reference from the examples provided by ST
Hello sir,
Iam using STM32L073Rz microcontrolller for flashing the data. But once we write the data in Particular address like 0X0802F000 iam not able to modify the string or numbers in the same address but we can flash another string in 0X0802F020 address. Please explain how to erase the previous flash data.
Iam trying to take external button as input after every press count value will be incremented and i am expecting to store the count value at any one address like 0X0802F000 after every increment count value stores at same address.
When use the board after power off also, count will be incremented from last value and it should be displayed on LCD or UART . Is it possible to do like that in Flash Programming.
I changed the GetPage function like this for STM32L073Rz is it correct
static uint32_t GetPage(uint32_t Address)
{
for (int indx=0; indx<512; indx++)
{
if((Address = (0x08000000 + 128*indx)))
{
return (0x08000000 + 128*indx);
}
}
return -1;
}
Please help to resolve the issue.
no it’s not correct. There is a lot confusion about the memory of the device that you have.
can u confirm the flash memory detail. does it have 1536 pages with each page being 128 bytes in size?
Yes sir,
Please verify in STM32L073RZ Referance Manual page no. 68/1034
RM0367 Reference manual
Hi there,
Is Bluepill board 64kb flash memory?
How do you use 128kb pages?
There is some confusion about 64 KB or 128 KB. But you can see in the video that it definitely uses the 128th page to write data, so I can say that it does have 128 KB of flash memory
Thanks for the reply and there is a confusion yes. But I thought it’s 64kb because it says 64kb in STM32CUBE IDE memory section on right bottom.